I recently spent some time making a custom autocomplete plug-in for Atom. There’s some good documentation out there for doing such a thing, but I found myself wanting more starter code. To put a programmer spin on an old saying, a code sample is worth a thousand words. So I created atom-autocomplete-boilerplate to help other developers hit the ground running.
Getting Started
In Atom parlance, a plug-in is called a package. Atom packages are written in JavaScript (or CoffeeScript, if you prefer). An autocomplete package contains one or more providers, which are the actual code objects that return suggestions. These providers work on top of Atom’s core autocomplete-plus package.
Atom provides a built-in way to download and install packages, but for local development, you’ll want to install atom-autocomplete-boilerplate differently.
- Clone the repo.
- Make sure you have apm installed.
- On Mac, you might need to start Atom and go to Atom > Install Shell Commands.
- On Windows, it’s probably already there.
- Open your terminal, navigate into the repo directory, then run
apm link
. - Back in Atom, you should now see atom-autocomplete-boilerplate installed.
- On Mac, you’ll find it under Atom > Preferences… > Packages > Community Packages.
- On Windows, you’ll find it under File > Settings > Packages > Community Packages.
Looking Around
Now’s a good time to open the atom-autocomplete-boilerplate project folder in Atom. Let’s take a tour!
package.json — Make sure you update this file with your own information! Also, notice this part at the end.
"engines": {
"atom": ">=1.15.0 <2.0.0"
},
"providedServices": {
"autocomplete.provider": {
"versions": {
"2.0.0": "getProvider"
}
}
}
You don’t need to change these values. engines
simply declares what versions of Atom are compatible. providedServices
lets Atom know that we’re using an autocomplete provider.
lib/main.js — Declares the providers.
lib/*-provider.js — These providers do the real work of providing suggestions. You’ll spend most of your time here. Detailed information is below.
data/*.json — Corresponding sources of suggestion data for each provider.
styles/styles.less — A place for custom CSS, if needed.
And lastly, you’ll notice a /samples folder. This isn’t necessary for the package, but it has some convenient sample files to test in.
Start Hacking
At this point I encourage you to start poking things. Maybe edit one of the data files to see different suggestions. Or sprinkle some console.log()
statements around to see what’s going on.
Console? Yep, Atom has dev tools, just like Chrome.
- On Mac, hit
option
+command
+I
. - On Windows, hit
ctrl
+shift
+I
.
One crucial note. When you make changes, you’ll need to reload Atom to see them take effect.
- On Mac, hit
ctrl
+option
+command
+L
. - On Windows, hit
ctrl
+shift
+F5
.
The Providers
The three included providers offer example code for an increasing number of features. For reference, here are the features that each one introduces.
lib/basic-provider.js — The absolute simplest provider you can make. Works off a simple array of words.
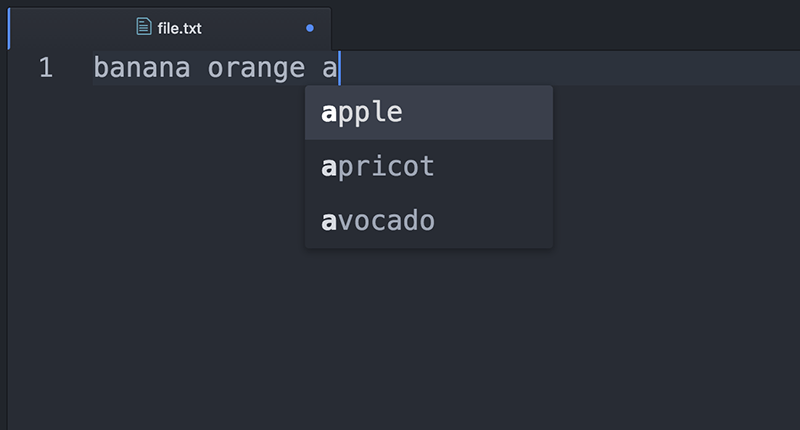
Features:
- Filters suggestions based on what was typed
lib/intermediate-provider.js — Takes things a little further. Reasonable starting point for a useful provider.
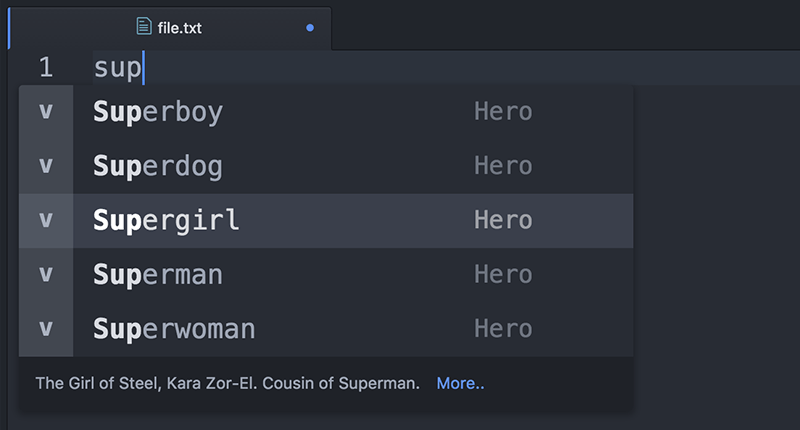
Features:
- Only offers suggestions when within certain file types
- Waits until 3 characters are typed before offering suggestions
- Case-insensitive suggestions
- Displays extra data along with suggestions: description, more link, type icon, right text label
lib/advanced-provider.js — Retrieves snippet suggestions from an external API asynchronously.
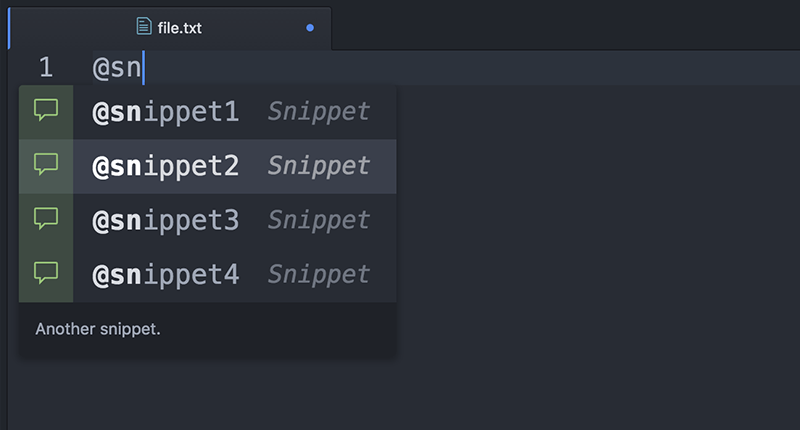
Features:
- Honors exceptions where suggestions will not be provided (within an HTML comment, in this case)
- Prioritizes its own suggestions above others
- Defines its own rules for determining the prefix used to find suggestions
- Uses JavaScript Promises to fetch suggestions asynchronously
- Retrieves suggestions from an external API instead of a local JSON file
- Suggests snippets (tokenized suggestions that allow users to fill in the blanks)
- Displays a custom icon next to suggestions
- Displays styled label text next to suggestions
- Fires an event handler after a suggestion is inserted
Have Fun
I like Atom’s autocomplete support. It’s reasonably engineered and gives you the freedom to do some pretty cool things. As you go on your way, here are some more references to help.
- Provider API — Documentation for creating a provider.
- Autocomplete Providers — List of providers that other people have created. Also has a handy list of grammar selectors (used when defining what file types a provider works with).