Let’s talk about making your site’s external links open in a new tab. First we’ll consider if this is even a good idea, then we’ll look at ways to improve the accessibility of these links.
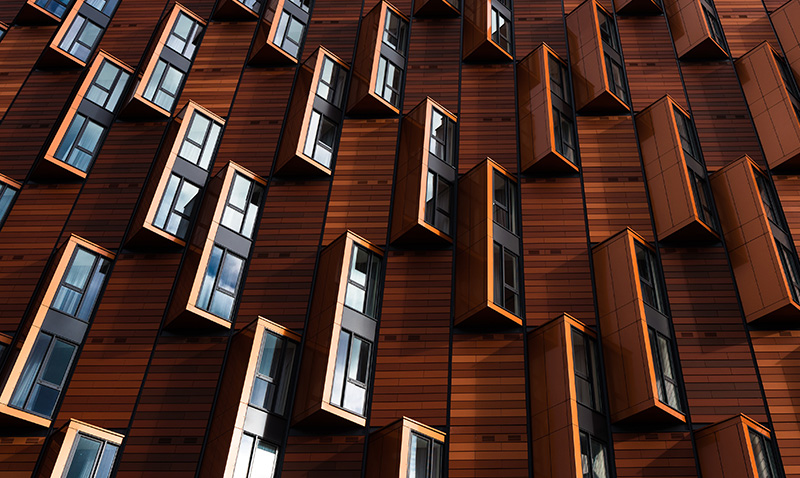
Should External Links Open in a New Tab?
Somewhere along the way, I learned to open external links in a new tab. If a link pointed to something not on the current domain, it got a target="_blank"
slapped on it. I know I’m not alone here — many of my fellow web developers do this. I thought it was convention.
But not everyone agrees. There are smart folks out there arguing against the idea, as you can see in this article by Chris Coyier and this article by Vitaly Friedman. They’ve got some good points, which I won’t rehash here. Instead, I want to focus on one particular reason to not open external links in a new tab: accessibility.
Accessibility Implications
With regards to accessibility, the W3C recommends against opening links in a new tab.
“In general, it is better not to open new windows and tabs since they can be disorienting for people, especially people who have difficulty perceiving visual content.”
Consider a user that doesn’t know/understand that they’re now in a different tab. They hit their trusty “back” keyboard shortcut to return to the previous page, but nothing happens. Not a great experience.
There are exceptions to the rule, though. In some situations, navigating the current tab can be very disruptive, in which case opening a new tab is preferable. For example, a link on a page with a long form could open in a new tab so the user doesn’t lose any data they’ve entered.
It’s also worth noting that the W3C’s advice against opening links in a new tab is expressed as an informative technique and not a success criteria. In other words, you can ignore this advice and still be fully WCAG 2.0 compliant. I don’t recommend you do that, though.
I’m Doing It Anyway
Alright, let’s assume you still want to open links in a new tab. Maybe you have one of those situations where a new tab is more accessible, as discussed earlier. Or maybe your boss/client is making you do it. Or maybe you just want to. I’m not here to judge, but I will help you make these links more accessible.
The important thing is to inform your users if a link will open in a new tab. This helps keep your site predictable, which is important for accessibility.
How To Inform Your Users
The most straightforward way is to put a message in the link text.
<a href="https://example.com" target="_blank">
example link (opens in a new tab)
</a>
If you don’t like the extra verbiage appearing in your links, then you can tweak things a bit to hide the message visually while keeping it available to screen readers (we’ll discuss adding a visual cue back in later in this article).
<a href="https://example.com" target="_blank">
example link
<span class="screen-reader-only">(opens in a new tab)</span>
</a>
The <span>
is styled to hide the text visually, but not from screen readers. There are a lot of ways to do this, but here’s one way.
.screen-reader-only {
position: absolute;
width: 1px;
clip: rect(0 0 0 0);
overflow: hidden;
white-space: nowrap;
}
Quick Security Note
This isn’t strictly accessibility related, but important enough to mention. Links that open in a new tab are vulnerable to a crafty exploit known as reverse tabnabbing (such a great name). Thankfully it’s easily prevented by setting rel="noopener"
on the link.
<a href="https://example.com" target="_blank" rel="noopener">
example link
</a>
Making Things Less Tedious
At this point, we’ve established that there’s some work to be done on links that open in a new tab in order to keep them accessible and safe. This can get tedious if you have a lot of links to worry about, so let’s have JavaScript do the work for us.
function addNoOpener(link) {
let linkTypes = (link.getAttribute('rel') || '').split(' ');
if (!linkTypes.includes('noopener')) {
linkTypes.push('noopener');
}
link.setAttribute('rel', linkTypes.join(' ').trim());
}
function addNewTabMessage(link) {
if (!link.querySelector('.screen-reader-only')) {
link.insertAdjacentHTML('beforeend', '<span class="screen-reader-only">(opens in a new tab)</span>');
}
}
document.querySelectorAll('a[target="_blank"]').forEach(link => {
addNoOpener(link);
addNewTabMessage(link);
});
The above code finds all links that open in a new tab, sets rel="noopener"
on them to protect against reverse tabnabbing, and inserts a hidden “opens in a new tab” message into each one for accessibility. Also, the code is idempotent, so your links will be in the correct state whether you run it one time or multiple times.
Adding a Visual Cue
We’ve got screen readers covered, but we still need a visual indication that a link will open in a new tab. If you decided to add a visible “opens in a new tab” message into your link text, then you’re already done. If not, then a common practice is to use an icon.
See the Pen New Tab Icon by Will Boyd (@lonekorean) on CodePen.
A little CSS is all you need to automatically add the icon to every link that opens in a new tab.
a[target="_blank"]::after {
content: url(new-tab-icon.svg);
display: inline-block;
margin-left: 0.2em;
width: 1em;
height: 1em;
}
Wrapping Up
If, like me, you always assumed that external links should open in a new tab, then maybe this article has given you some food for thought. I’m not trying to say that new tabs are evil, but there is more to consider when you use them, especially with regards to accessibility.