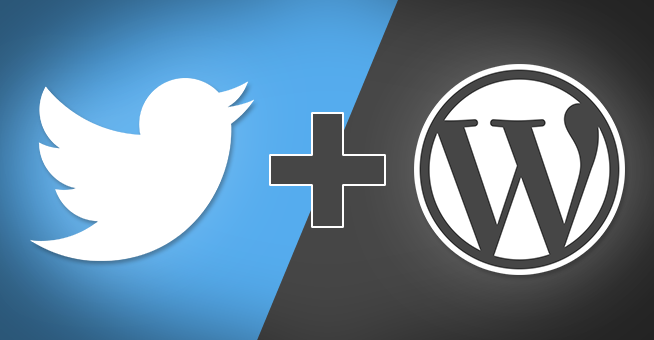
Last time I gave you the rundown on how to use Twitter Cards. I covered everything you needed to manually add the markup to your pages, but if you run a WordPress blog (like this one), you probably want something more automated.
Of course, there are WordPress plugins to do this for you. I was using one for a while, but then decided to brew my own solution. Nothing against plugins, but it was overkill for my needs. Plus, it was an excuse to learn something new.
The Meta Tags
Add this code to header.php (inside of <head>
):
<?php if (is_single()) :
$p = get_queried_object();
$card_title = htmlspecialchars($p->post_title);
$card_description = htmlspecialchars(get_card_description($p->ID));
$card_image = htmlspecialchars(get_card_image($p->ID));
?>
<meta name="twitter:card" content="summary_large_image">
<meta name="twitter:creator" content="@lonekorean">
<meta name="twitter:site" content="@lonekorean">
<meta name="twitter:title" content="<?php echo $card_title; ?>">
<meta name="twitter:description" content="<?php echo $card_description; ?>">
<?php if(!empty($card_image)) : ?>
<meta name="twitter:image" content="<?php echo $card_image; ?>">
<?php endif; ?>
<?php endif; ?>
A quick is_single()
check makes sure we only do this stuff on a post page. After that, we gather up some data and spit it out in meta tags. Some of these values (card
, creator
, site
) won’t change post to post, so we can hardcode them. The rest need to be pulled from the post data.
Getting title
is easy. description
and image
would be easy, too… if we were inside The Loop. Sadly, we’re not. No biggie, we’ll just have to work harder with less convenient functions.
The Support Functions
Add this code to functions.php:
function get_card_description($post_id) {
$desc = get_post($post_id)->post_content;
// remove junk and collapse whitespace
$desc = trim(strip_tags(strip_shortcodes($desc)));
$desc = preg_replace('/\s+/', ' ', $desc);
// trim to size
preg_match('/^.{0,199}\S\b/', $desc, $matches);
$desc = $matches[0] . '...';
return $desc;
}
function get_card_image($post_id) {
$src = get_the_post_thumbnail($post_id);
// extract src out of html
if ($src != '' && preg_match('/src="(.*?)"/', $src, $matches)) {
$src = $matches[1];
}
return $src;
}
I use the first 200 characters of the post content for description
. The get_card_description()
function takes care of all the text massaging and trimming to make this happen. If you’re curious about the regex on line 9, read this post.
When creating a post, I set the post’s featured image to whatever I want to see in image
. The get_card_image()
function retrieves the image URL for me to use.
Troubleshooting Featured Image
If you don’t see the ability to set a featured image on the post add/edit page, then click the “Screen Options” tab at the top right of the page and make sure the “Featured Image” checkbox is ticked. If you don’t see a checkbox for “Featured Image”, then you probably need to add featured image support to your WordPress theme. Don’t worry, it’s easy. Just add something like this to your functions.php:
function mytheme_setup() {
add_theme_support( 'post-thumbnails' );
}
add_action( 'after_setup_theme', 'mytheme_setup' );
Done
And that’s it. Next time you publish a post, the Twitter Card markup will be set for you. Just make sure you set a featured image for the post if you want one to show in the Twitter Card.